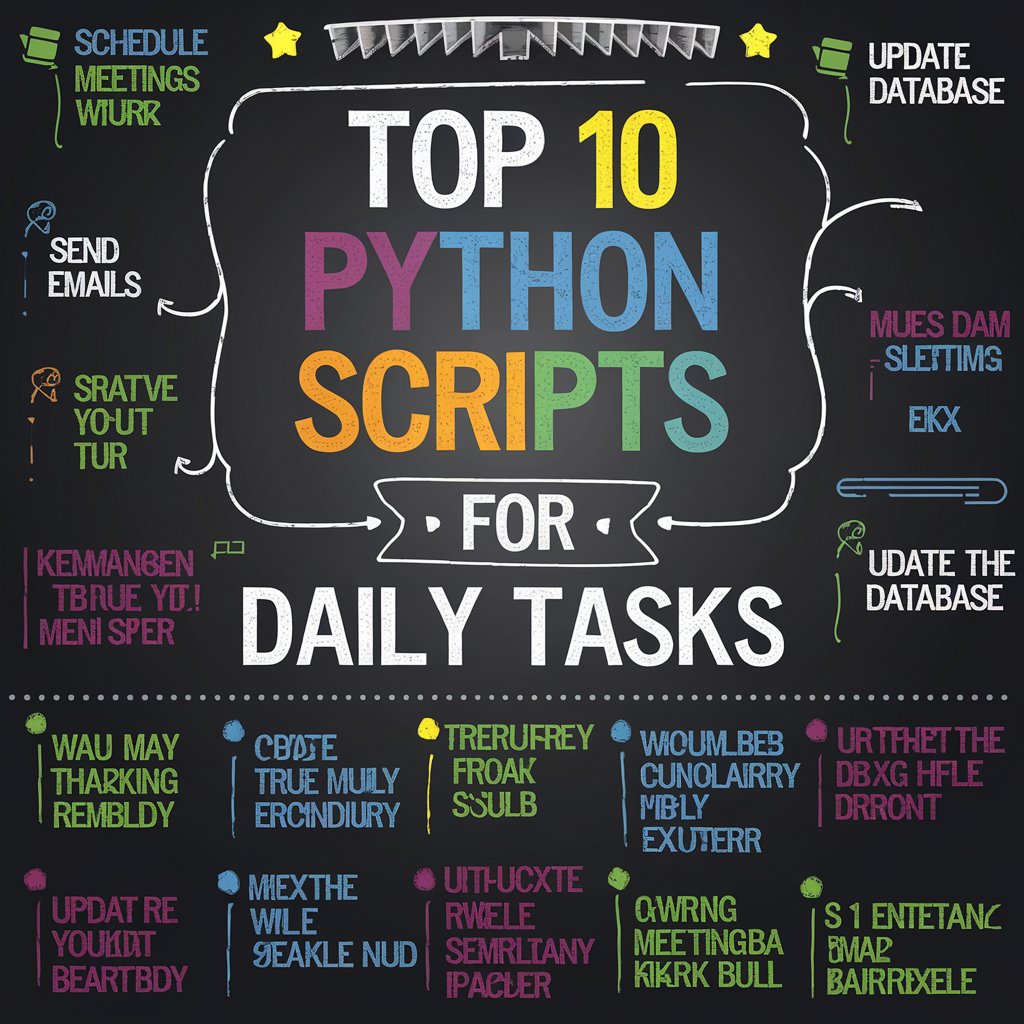
Top 10 Python Useful Script for daily task
In this article, we will explore 10 Python scripts that can make your task automatic.
1. Automate Email Sending
- Use Python to send emails with attachments.
- Libraries: smtplib, email
import smtplib
from email.mime.text import MIMEText
def send_email(subject, body, to_email):
smtp_server = "smtp.gmail.com"
smtp_port = 587
sender_email = "your_email@gmail.com"
sender_password = "your_password"
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = sender_email
msg['To'] = to_email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(sender_email, sender_password)
server.sendmail(sender_email, to_email, msg.as_string())
2. Web Scraping for Data Extraction
- Automate data extraction from websites.
- Libraries: requests, BeautifulSoup
import requests
from bs4 import BeautifulSoup
def scrape_weather():
url = "https://weather.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.string)
3. File Rename
- This script is handy when you need to rename multiple files in a folder based on specific criteria. For example, you can add a prefix, suffix, or replace text in filenames.
- Libraries: OS
import os
folder_path = '/path/to/folder'
for filename in os.listdir(folder_path):
if filename.startswith('prefix_'):
new_filename = filename.replace('prefix_', 'new_prefix_')
os.rename(os.path.join(folder_path, filename), os.path.join(folder_path, new_filename))
4. Automate Currency Conversion
- Convert currencies using APIs.
- Libraries: ExchangeRate-API
import requests
# Your API key from ExchangeRate-API
api_key = 'YOUR_API_KEY'
# Base currency and target currency
base_currency = 'USD' # Change this to your base currency (e.g., 'USD', 'EUR')
target_currency = 'EUR' # Change this to the currency you want to convert to (e.g., 'EUR', 'GBP')
# The amount you want to convert
amount_to_convert = 100 # Change this to the amount you want to convert
# URL to get the currency rates
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/{base_currency}"
# Make a GET request to the API
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Check if the target currency is available in the response
if target_currency in data['conversion_rates']:
# Get the conversion rate for the target currency
conversion_rate = data['conversion_rates'][target_currency]
# Convert the amount
converted_amount = amount_to_convert * conversion_rate
print(f"{amount_to_convert} {base_currency} = {converted_amount:.2f} {target_currency}")
else:
print(f"Error: {target_currency} not found in conversion rates.")
else:
print("Error: Unable to fetch data from the API.")
How It Works:
- API Key: You need to replace
YOUR_API_KEY
with your actual API key from ExchangeRate-API. - Base Currency: Set the base currency you want to convert from (e.g.,
USD
). - Target Currency: Set the currency you want to convert to (e.g.,
EUR
). - Amount: Set the amount you want to convert.
5. Data Backup Script
- Automate the backup of files and directories to ensure data safety:
- Libraries: shutil
import shutil
def create_backup(source_dir, backup_file):
shutil.make_archive(backup_file, 'zip', source_dir)
6. Data Analysis with Pandas
- Pandas is a powerful library for data analysis and manipulation. data from various sources like CSV files or databases.
import pandas as pd
# Read data from a CSV file
data = pd.read_csv('data.csv')
# Perform basic analysis
mean = data['column_name'].mean()
print(f"Mean: {mean}")
7. Automate Text Translation
- Translate text using APIs.
- Libraries: googletrans
from googletrans import Translator
def translate_text(text, dest_lang):
translator = Translator()
return translator.translate(text, dest=dest_lang).text
8. Automate PDF Manipulation
- Merge, split, or extract text from PDFs.
- Libraries: PyPDF2
from PyPDF2 import PdfReader, PdfMerger
def merge_pdfs(pdf_list, output):
merger = PdfMerger()
for pdf in pdf_list:
merger.append(pdf)
merger.write(output)
9. Automate Image Processing
- Resize, rotate, or add watermarks.
- Libraries: Pillow
from PIL import Image
def resize_image(image_path, output_path, size):
with Image.open(image_path) as img:
img.resize(size).save(output_path)
10. Automate Database Backups
- Backup databases like MySQL.
- Libraries: subprocess
import subprocess
def backup_mysql(user, password, db_name, output):
cmd = f"mysqldump -u {user} -p{password} {db_name} > {output}"
subprocess.run(cmd, shell=True)